Convert bitbucket pipeline test reports into pull requests code insight reports
Platform Notice: Cloud Only - This article only applies to Atlassian products on the cloud platform.
Summary
When a build step generates test results, pipelines will automatically detect and show any failures in the web interface. This works with JUnit and Maven Surefire XML formats, regardless of the language they are written in, as explained in this document. But test report summaries are not automatically updated with the commit.
Solution
Code insights Bitbucket rest APIs provide reports, annotations, and metrics to help you and your team improve code quality in pull requests throughout the code review process. Some of the available code insights are static analysis reports, security scan results, artifact links, unit tests, and build status. Please refer to this document to learn more about Code insights reporting APIs.
One can leverage Code insights Bitbucket rest APIs to download and update the test report summary link to a commit, which will show the reports under relevant pull requests.
Example:
Step 1: Run your tests, store the BITBUCKET_STEP_UUID of the test step in a variable, as shown below, and export it as an artifact.
1
2
3
4
5
6
7
8
9
10
pipelines:
default:
- step:
name: Tests
script: # Modify the commands below to build your repository.
- export IMAGE_NAME2=easyad/easyad_nginx:$BITBUCKET_COMMIT
- /bin/bash bitbucket_pipeline_tests.sh
- echo ${BITBUCKET_STEP_UUID} >> step.txt
artifacts:
- step.txt
Step 2: Fetch the test report summary from the previous pipeline step using the reports API, and extract test report summary values as a variable.
1
2
3
4
5
6
7
8
9
10
11
- step:
name: get the test report summary from pipleline step
script: # Modify the commands below to build your repository.
- step=$(cat step.txt)
- apt install jq
- output=$(curl -vvvv --location -g -u "${USERNAME}:${MY_APP_PASSWORD}" --request GET "https://api.bitbucket.org/2.0/repositories/${BITBUCKET_WORKSPACE}/${BITBUCKET_REPO_SLUG}/pipelines/${BITBUCKET_PIPELINE_UUID}/steps/${step}/test_reports")
- echo $output
- number_of_test_cases=$(echo ${output} | jq -r '.number_of_test_cases')
- number_of_failed_test_cases=$(echo ${output} | jq -r '.number_of_failed_test_cases')
- number_of_successful_test_cases=$(echo ${output} | jq -r '.number_of_successful_test_cases')
- if [ "${number_of_failed_test_cases}" -eq "0" ];then export status_report=PASSED; else export status_report=FAILED; fi
Step 3: Update the extracted values as a test summary report and update them to the ${BITBUCKET_COMMIT} using Code insights Bitbucket rest API.
Sample python script:
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
import requests
import json
import os
password = os.environ.get("MY_APP_PASSWORD")
username = os.environ.get("USERNAME") #"USERNAME" is bitbucket username.
url = "https://api.bitbucket.org/2.0/repositories/${BITBUCKET_WORKSPACE}/${BITBUCKET_REPO_SLUG}/commit/${BITBUCKET_COMMIT}/reports/mysystem-001"
headers = {
"Accept": "application/json",
"Content-Type": "application/json",
"Authorization": "basic_auth(username, password)"
}
payload = json.dumps( {
"title": "TEST",
"details": "This pull request introduces new dependency vulnerabilities.",
"report_type": "TEST",
"result": os.environ.get("status_report"),
"reporter": "mySystem",
"data": [
{
"title": "No of Test Cases",
"type": "NUMBER",
"value": os.environ.get("number_of_test_cases")
},
{
"title": "Failed Tests",
"type": "NUMBER",
"value": os.environ.get("number_of_failed_test_cases")
},
{
"title": "Successful Tests",
"type": "NUMBER",
"value": os.environ.get("number_of_successful_test_cases")
}
]
} )
response = requests.request(
"PUT",
url,
data=payload,
headers=headers
)
print(json.dumps(json.loads(response.text), sort_keys=True, indent=4, separators=(",", ": ")))
Results
Sample test summary report linked to the pull-request source commit under the Pull Requests Reports section:
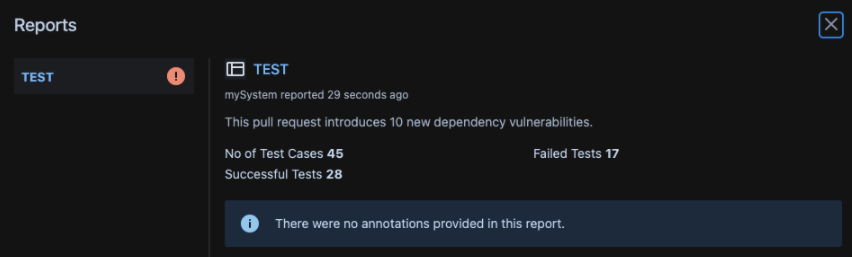
Was this helpful?